Here is the Query to select the top N records from Access database's table:
SELECT Top N Table.*FROM Table where Column is not null;
Wednesday, December 28, 2011
Monday, October 31, 2011
A network-related or instance-specific error occurred while establishing a connection to SQL Server.
Problem: A network-related or instance-specific error occurred while establishing a connection to SQL Server.
Server OS: windows 2008
Client OS: Windows 7
Solution: Open outbound port on client machine, and open inbound port on server
1.On Server - Open outbound port by adding a new rule for port 1433 in firewall at server machine
2.On Client - Open inbound port by adding a new rule for port 1433 in firewall at client machine
See the sample "How to add rule for inbound/outbound port"
Server OS: windows 2008
Client OS: Windows 7
Solution: Open outbound port on client machine, and open inbound port on server
1.On Server - Open outbound port by adding a new rule for port 1433 in firewall at server machine
2.On Client - Open inbound port by adding a new rule for port 1433 in firewall at client machine
See the sample "How to add rule for inbound/outbound port"
Tuesday, October 25, 2011
Alternate of IssApp command in windows 2008
This is a shortcut alternate for IssApp command in windows 2008:
Go to C:\Windows\system32 and type inetsrv\appcmd.exe list wp
Here is the output you will get for this command:
WP "7056" (applicationPool:1c931ee15fb748979d16454c705d8d07)
WP "11392" (applicationPool:SecurityTokenServiceApplicationPool
WP "3956" (applicationPool:SharePoint - 80)
Go to C:\Windows\system32 and type inetsrv\appcmd.exe list wp
Here is the output you will get for this command:
WP "7056" (applicationPool:1c931ee15fb748979d16454c705d8d07)
WP "11392" (applicationPool:SecurityTokenServiceApplicationPool
WP "3956" (applicationPool:SharePoint - 80)
Tuesday, October 4, 2011
How to change owner of Sharepoint site from administrator to NetworkService
Steps to "How to change owner of Sharepoint site from administrator to NetworkService":
A. Look application pools in IIS
- Look the identity column in application pool List in IIS 6.0 and the select the application pool which is using administrator as Identity.
- Change identity from Administrator to NetworkService(right click on a pool, click Advance setting, click on Identity and type NetworkService)
B. Open Database server where Sharepoint database is created for central admin as well as content dbs for webApps(Login to db server with Administrative or DomAdminitrator’s userId)
- Go to security and select user NetworkService. Right click and select properties.
- Allow access for this user as dbo by selecting User Mapping. User mapping will show you all the database for this userid.
- Click on each database name and select db_owner under “database role membership”
- If your content database was offline or under suspect condition, make it online now.
C. Restart IIS
Open Central admin or any sharepoint site now for testing
Thursday, September 29, 2011
search SharePoint list and return matching column using LINQ
I Have writen this reusable code, which will open a Sharepoint List, and search that list and return the first matching row's specific column:
This function need 04 parameters: ListName, Search FieldName, Search Value, and return Column name.
If you like this solution, then care for a tree which need some water, so give water to a plant and make that green.
Start using http://www.code4green.com as your free code generation tool for Sharepoint, PowerShell, C#, ASP.net, SQL, and many more languages.
let other know about this tool, because this tool is helping community.
This function need 04 parameters: ListName, Search FieldName, Search Value, and return Column name.
public string GetAColumnValueFromList(string listName, string Field, string value, string returnField)
{
string outData = string.Empty;
DataTable dtResult = new DataTable();
try
{
if (oList == null)
{
using (SPSite oSiteCollection = new SPSite(siteUrl))
{
using (SPWeb oWebsiteRoot = oSiteCollection.OpenWeb("/"))
{
oList = oWebsiteRoot.Lists[listName];
}
}
}
List items = (from li in oList.Items.OfType()
where li["GUID1"].ToString() == value
select li).ToList();
foreach (SPListItem item in items)
{
outData = item[returnField].ToString();
}
return outData;
}
catch (Exception ex)
{
EnterpriseLibrary.HandleException(ex, Policies.BLL);
throw ex;
}
}
If you like this solution, then care for a tree which need some water, so give water to a plant and make that green.
Start using http://www.code4green.com as your free code generation tool for Sharepoint, PowerShell, C#, ASP.net, SQL, and many more languages.
let other know about this tool, because this tool is helping community.
LINQ Query on SPListItem returning "Value does not fall within the expected range."
If you are doing LINQ Qery on SHarepoint SpListItem object and getting the following error: "Value does not fall within the expected range."
Might be this little simple (addition of .ToString()) will help you to make it work.
Original Code
List items = (from li in oList.Items.OfType()
where li["UserName"] == value
select li).ToList();
change this query to like this by adding .ToString() in li["ColName"]=="Value"
Changed Code
List items = (from li in oList.Items.OfType()
where li["Username"].ToString() == value
select li).ToList();
If you like this solution, then care for a tree which needs some water, so water a plant and make that green.
Start using http://www.code4green.com as your free code generation tool for Sharepoint, PowerShell, C#, ASP.net, SQL, and many more languages.
let other know about this tool, because this tool is helping community to plant tree.
Might be this little simple (addition of .ToString()) will help you to make it work.
Original Code
List
where li["UserName"] == value
select li).ToList
change this query to like this by adding .ToString() in li["ColName"]=="Value"
Changed Code
List
where li["Username"].ToString() == value
select li).ToList
If you like this solution, then care for a tree which needs some water, so water a plant and make that green.
Start using http://www.code4green.com as your free code generation tool for Sharepoint, PowerShell, C#, ASP.net, SQL, and many more languages.
let other know about this tool, because this tool is helping community to plant tree.
Monday, September 19, 2011
Windows service is not able to read outlook email
Problem:
If you are trying to read the outlook email (using outlook api) from your "windows service", then it will not show any email.
Reason: Your service is running under Local System Account, which has no profile in your outlook.
Your service will runn success full but it will not show any email because of not-matched user profile.
Solution: Change the service account "Log On" option, so your windows service will run under a user account which has user profile for outlook.
Steps: Here are the steps to fix this issue -
1. Right click on My Computer, click on Manage
2. Clicke on Service, and select your service like "Code4Green Email Reader"
3. Click on service properties
4. Click the tab Log On
5. select Logon as: This account -
6. enter outlook's profile login name with domain name
7. enter password
8. enter confirm password
9. click Apply
10. click OK
Reason: Your service is running under Local System Account, which has no profile in your outlook.
Your service will runn success full but it will not show any email because of not-matched user profile.
Solution: Change the service account "Log On" option, so your windows service will run under a user account which has user profile for outlook.
Steps: Here are the steps to fix this issue -
1. Right click on My Computer, click on Manage
2. Clicke on Service, and select your service like "Code4Green Email Reader"
3. Click on service properties
4. Click the tab Log On
5. select Logon as: This account -
6. enter outlook's profile login name with domain name
7. enter password
8. enter confirm password
9. click Apply
10. click OK
Friday, September 16, 2011
Remove the dll from GAC without using GAC util
Using the following name space :System.EnterpriseServices.Internal, you can uninstall or remove assembly from GAC without using GacUril in your production environment.
I have seen on web, that people are getting hard time to use Publish.GacRemove method, because they don't know how to give the path name.
Here are two step to uninstall the dll from GAC without using GacUtil.
STEP 1:
Copy the existing dll into a temp folder and then use that path to remove/uninstall. Here is the Powershell code to copy the dll from gac to directory:
dir C:\Windows\Assembly -Recurse -Filter "YourDLLName*.dll" foreach { copy $_.FullName C:\Temp\assemblies }
STEP 2:
Here is the powershell code to remove the dll from gac using path in step1
# load System.EnterpriseServices assembly
[Reflection.Assembly]::LoadWithPartialName("System.EnterpriseServices, Version=2.0.0.0")
# create an instance of publish class
[System.EnterpriseServices.Internal.Publish] $publish = new-object System.EnterpriseServices.Internal.Publish
#UnInstall UserTracking.DAL.dll in gac
$AssemblyFullName = "C:\Temp\Assembly\YourDLLName*.dll"
$publish.GacRemove($AssemblyFullName)
dll name in $AssemblyFullName = "C:\Temp\Assembly\YourDLLName*.dll", is copied from GAC to C:\Temp\Assembly folder in step1.
I hope this article will help to someone, please plant a tree in your life span if you get any help from this as a donation to www.code4green.com
I have seen on web, that people are getting hard time to use Publish.GacRemove method, because they don't know how to give the path name.
Here are two step to uninstall the dll from GAC without using GacUtil.
STEP 1:
Copy the existing dll into a temp folder and then use that path to remove/uninstall. Here is the Powershell code to copy the dll from gac to directory:
dir C:\Windows\Assembly -Recurse -Filter "YourDLLName*.dll" foreach { copy $_.FullName C:\Temp\assemblies }
STEP 2:
Here is the powershell code to remove the dll from gac using path in step1
# load System.EnterpriseServices assembly
[Reflection.Assembly]::LoadWithPartialName("System.EnterpriseServices, Version=2.0.0.0")
# create an instance of publish class
[System.EnterpriseServices.Internal.Publish] $publish = new-object System.EnterpriseServices.Internal.Publish
#UnInstall UserTracking.DAL.dll in gac
$AssemblyFullName = "C:\Temp\Assembly\YourDLLName*.dll"
$publish.GacRemove($AssemblyFullName)
dll name in $AssemblyFullName = "C:\Temp\Assembly\YourDLLName*.dll", is copied from GAC to C:\Temp\Assembly folder in step1.
I hope this article will help to someone, please plant a tree in your life span if you get any help from this as a donation to www.code4green.com
Monday, June 13, 2011
Best way to handle null in C# - Use Null Coalescing Operator
The Best way to handle null in C# - Use Null Coalescing Operator. You have seen some samples where I have used ?? operator, that is nothing but a "Null Coalescing Operator"
Null Coalescing Operator or ?? Operator in C# is supported in Visual Studio 2005, 2008, and 2010.
The Null Coalescing Operator or ?? operator returns the left-hand operand if it is not null, or else it returns the right operand.
string strData;
strData = rsDB("FieldName").ToString() ?? "No Data";
strData will get value "No Data" in case of rsDB("FieldName") is null.
Enjoy Green coding @ www.Code4Green.com
Null Coalescing Operator or ?? Operator in C# is supported in Visual Studio 2005, 2008, and 2010.
The Null Coalescing Operator or ?? operator returns the left-hand operand if it is not null, or else it returns the right operand.
string strData;
strData = rsDB("FieldName").ToString() ?? "No Data";
strData will get value "No Data" in case of rsDB("FieldName") is null.
Enjoy Green coding @ www.Code4Green.com
Tuesday, May 24, 2011
The security validation for this page is invalid. Click Back in your Web browser, refresh the page, and try your operation again.
If you are getting the following error while Attaching a EventHandler on a Sharepoint List, than follow these three steps to resolve that issue..
Error: The security validation for this page is invalid. Click Back in your Web browser, refresh the page, and try your operation again.
Solution: Add the following Lines in you List Update code:
1. SPSecurity.RunWithElevatedPrivileges(delegate(){ - See Line 01
2. Add site.RootWeb.AllowUnsafeUpdates = true; , See Line 04
3. Add site.WebApplication.FormDigestSettings.Enabled = false;, See line 10
Sample Code
SPSecurity.RunWithElevatedPrivileges(delegate(){
using (SPSite site = new SPSite(URL))
{
site.RootWeb.AllowUnsafeUpdates = true;
using (SPWeb siteWeb = site.OpenWeb())
{
SPList list = siteWeb.Lists["TestList"];
SPEventReceiverDefinition defItemAdding = list.EventReceivers.Add();
site.WebApplication.FormDigestSettings.Enabled = false;
defItemAdding.Assembly = "WSPBuilderProject1, Version=1.0.0.0, Culture=neutral, PublicKeyToken=e0a58138cf93116a";
defItemAdding.Class = "WSPBuilderProject1.TestListEH";
defItemAdding.Name = "AddingEventHandler";
defItemAdding.Type = SPEventReceiverType.ItemAdding;
defItemAdding.SequenceNumber = 1001;
//defItemAdding.Synchronization = SPEventReceiverSynchronization.Synchronous;
defItemAdding.Update();
site.WebApplication.FormDigestSettings.Enabled = true;
}
site.RootWeb.AllowUnsafeUpdates = false;
}
});
Enjoy Sharepoint Green Coding @ Code4Green.com
Error: The security validation for this page is invalid. Click Back in your Web browser, refresh the page, and try your operation again.
Solution: Add the following Lines in you List Update code:
1. SPSecurity.RunWithElevatedPrivileges(delegate(){ - See Line 01
2. Add site.RootWeb.AllowUnsafeUpdates = true; , See Line 04
3. Add site.WebApplication.FormDigestSettings.Enabled = false;, See line 10
Sample Code
SPSecurity.RunWithElevatedPrivileges(delegate(){
using (SPSite site = new SPSite(URL))
{
site.RootWeb.AllowUnsafeUpdates = true;
using (SPWeb siteWeb = site.OpenWeb())
{
SPList list = siteWeb.Lists["TestList"];
SPEventReceiverDefinition defItemAdding = list.EventReceivers.Add();
site.WebApplication.FormDigestSettings.Enabled = false;
defItemAdding.Assembly = "WSPBuilderProject1, Version=1.0.0.0, Culture=neutral, PublicKeyToken=e0a58138cf93116a";
defItemAdding.Class = "WSPBuilderProject1.TestListEH";
defItemAdding.Name = "AddingEventHandler";
defItemAdding.Type = SPEventReceiverType.ItemAdding;
defItemAdding.SequenceNumber = 1001;
//defItemAdding.Synchronization = SPEventReceiverSynchronization.Synchronous;
defItemAdding.Update();
site.WebApplication.FormDigestSettings.Enabled = true;
}
site.RootWeb.AllowUnsafeUpdates = false;
}
});
Enjoy Sharepoint Green Coding @ Code4Green.com
Tuesday, May 3, 2011
How To Add Windows Live Messanger in your website?

If you know HTML, than you can do this easily, just follow these 12 steps and see these steps in attached screen shot. I am sure on the 12th step, you will see the miracle... :)
1. Login to http://login.live.com with you live/hotmail/msn email id
2. go to setting page Windows Live Setting
3. click on WebSetting link in left side menu
4. Select checkbox for " Allow anyone on the web to see my presence and send me messages."
5. Click Save button on top of the page
6. Click on Create HTML link in left side menu
7. Choose which control you want to display on your page. It has three options
7.1 - IM window - It embed the messanger as a frame. It will allow chating within your site's page. This will give you the option to
select height, width and theme color for your messanger.
7.2 - Button - Show button with current status of messanger. This will launch the web based windows IM in new window.
7.3 - Status Icon -> This will show only status icon of your messanger's status. This will launch the web based windows IM in new
window.
NOTE: Step 7.1, and 7.2 will open a new page with the following url with your Live Invite Id as invitee:
http://settings.messenger.live.com/Conversation/IMMe.aspx?invitee=bd72eb1303b8b293@apps.messenger.live.com&mkt=en-CA
8. After selecting the option in step 7, it will generate the html and display in textbox next to label "Copy the HTML and paste it into
your webpage"
8.1. copy and paste html in your html or asp.net page and run it.
9. Once you will run your page, than it will ask a visitor to begin conversation. Click on Begin Conversation link.
10. Onclick over Begin Conversation, it will popup a window to type visitor's name. So type your name here and hit OK button.
11. After entering your name as visitor, system will popup Captcha screen, enter the characters shown on the image 2gNC3V0g and click on
submit button.
12. Now you are ready to chat, start doing chating.
Enjoy your green code @code4green.com
1. Login to http://login.live.com with you live/hotmail/msn email id
2. go to setting page Windows Live Setting
3. click on WebSetting link in left side menu
4. Select checkbox for " Allow anyone on the web to see my presence and send me messages."
5. Click Save button on top of the page
6. Click on Create HTML link in left side menu
7. Choose which control you want to display on your page. It has three options
7.1 - IM window - It embed the messanger as a frame. It will allow chating within your site's page. This will give you the option to
select height, width and theme color for your messanger.
7.2 - Button - Show button with current status of messanger. This will launch the web based windows IM in new window.
7.3 - Status Icon -> This will show only status icon of your messanger's status. This will launch the web based windows IM in new
window.
NOTE: Step 7.1, and 7.2 will open a new page with the following url with your Live Invite Id as invitee:
http://settings.messenger.live.com/Conversation/IMMe.aspx?invitee=bd72eb1303b8b293@apps.messenger.live.com&mkt=en-CA
8. After selecting the option in step 7, it will generate the html and display in textbox next to label "Copy the HTML and paste it into
your webpage"
8.1. copy and paste html in your html or asp.net page and run it.
9. Once you will run your page, than it will ask a visitor to begin conversation. Click on Begin Conversation link.
10. Onclick over Begin Conversation, it will popup a window to type visitor's name. So type your name here and hit OK button.
11. After entering your name as visitor, system will popup Captcha screen, enter the characters shown on the image 2gNC3V0g and click on
submit button.
12. Now you are ready to chat, start doing chating.
Enjoy your green code @code4green.com
Thursday, April 28, 2011
Create a new SharePoint column in User Profile's list
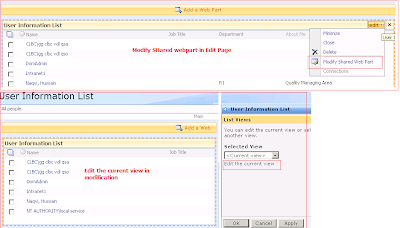
How to get a hidden Sharepoint List for User Profile?
Here is the way to find out hidden user profile's list in sharepoint: At the top level site in site collection, type this url: /_catalogs/users/simple.aspx , that will give you User Information List's default view.
Here is the way to find out hidden user profile's list in sharepoint: At the top level site in site collection, type this url: /_catalogs/users/simple.aspx , that will give you User Information List's default view.
Go and edit this page(site action >> edit page), and than modifiy Shared webpart. It will give list view of this list, edit the current view. Edit will show the editable view of that user Profile's hidden list.
Now you got the place where you can see all the column from this hidden treasure, now it is the time to add a new column in this list, so you can use this column in your custom permission's code:
Here is the PowerShell to Create a New Coloumn in hidden site collection level's "User Information List"
[system.reflection.assembly]::loadwithpartialname("microsoft.sharepoint")
$site= New-Object Microsoft.SharePoint.SPSite ("http://YourSiteName")
$web=$site.OpenWeb()
$list=$web.Lists["User Information List"]
$list.Fields.Add("Region1", "Text", 0)
$site= New-Object Microsoft.SharePoint.SPSite ("http://YourSiteName")
$web=$site.OpenWeb()
$list=$web.Lists["User Information List"]
$list.Fields.Add("Region1", "Text", 0)
Tuesday, April 19, 2011
WebMatrix - New Buzzword in IT Industry
WebMatrix is a free tool that allows you to create, customize and publish websites, Believe me It’s amazingly easy to use.
WebMatrix connects you to a world of popular and free open-source Web applications, including WordPress, DotNetNuke, Umbraco, Joomla!, Moodle, Drupal , and many more(till now 49 apps). You can select a website from built-in gallery, and WebMatrix will handle all the downloading and installation of your new Web site.
Here is the list of Features of WebMatrix which makes it very powerful and #1 development tool for the web development.
Features List of WebMatrix
WebMatrix connects you to a world of popular and free open-source Web applications, including WordPress, DotNetNuke, Umbraco, Joomla!, Moodle, Drupal , and many more(till now 49 apps). You can select a website from built-in gallery, and WebMatrix will handle all the downloading and installation of your new Web site.
Here is the list of Features of WebMatrix which makes it very powerful and #1 development tool for the web development.
Features List of WebMatrix
- Small, but complete package - It is free web development tool. It is small in size but very powerful tool.
- Desktop based Web Development and Testing Environment - You will get the same powerful Web server(IIS Express), database engine(SQL CE4), and frameworks(MVC 3.0) that your Web site on the internet uses. This ensures that your transition from development to production is smooth and seamless.
- Easy to Code - Start with HTML, CSS and JavaScript and then seamlessly connect to a database or add in dynamic server code using the new ‘Razor’ syntax for ASP.NET Web pages.
- Webmatrix have intellisense - it also supports tag completion and hinting for standard HTML and CSS markup making it easier and faster for you to write code.
- Simple Publishing - You can find host company to suite your need. Use webMatrix to find your web host which supports webmatrix.
- Easy to cross bowser testing - You can test your site directly will diffrent browsers using webmartix tool.
- Last but not least - It will connect you with lots of open source web applications(till the date i am writing this blog - 49 applications incliding most populars WordPress, DotNetNuke, Umbraco, Joomla!, Moodle, Drupal and more)
- Make your site More Search engine friendly - Run an SEO report and find how to make your site more visible to search engines.
- Code Helper is the best helping tool - Want to display a Twitter feed? Need to show a video? Code helpers make common tasks easy to do with just a simple tag in your HTML(Yes it is HTML not server side...).
- Nice Interface with IDE, Database, IIS, FTP, and more - WebMatrix has rich code editor, a database editor, Web server management, Search Engine Optimization, FTP publishing, and many more..
- Easy and Lightweight Database - Using a database has never been easier! WebMatrix includes a small, embedded database SQL Server CE 4. Copy that database from your machine to web server under bin folder and you are done.
Enjoy your Green coding @ code4Green.com
Thursday, April 14, 2011
Compare powershell and STSAdm commands for SharePoint

Here is the Most important STSAdm commands which is used in Sharepoint 2007 for scripting. I have converted those comands into PowerShell, so you can compare and use it. Here is the sample to compare side by side STSAdm and PowerShell commands for Sharepoint:
- STSAdm Command
- stsadm –o addsolution –filename “D:\Temp\YourSharePointSolution.wsp“
- stsadm –o deploysolution –name YourSharePointSolution.wsp –url http://myspwebappp –allowgacdeployment –immediate
- stsadm –o upgradesolution –name YourSharePointSolution.wsp –filename “D:\Temp\YourSharePointSolution.wsp” -immediate
- stsadm –o retractsolution –name YourSharePointSolution.wsp –url http://myspwebapp –immediate
- stsadm –o deletesolution –name YourSharePointSolution.wsp
- stsadm –o activatefeature –name YourFeatureName –url http://YourSharePointWebApp
- stsadm –o deactivatefeature –name YourFeatureName –url http://YourSharePointWebApp
- stsadm -o execadmsvcjobs
- stsadm -o userrole -userlogin "YourUserOrGroupName" -role "Approve" -add -url "http:/YourSPSite"
- PowerShell Command
- Add-SPSolution “D:\Temp\YourSharePointSolution.wsp“
- Install-SPSolution –Identity YourSharePointSolution.wsp –WebApplication http://myspwebapp –GACDeployment
- Update-SPSolution –Identity YourSharePointSolution.wsp –LiteralPath “D:\Temp\YourSharePointSolution.wsp” –GacDeployment
- Uninstall-SPSolution –Identity YourSharePointSolution.wsp –WebApplication http://myspwebapp
- Remove-SPSolution–Identity YourSharePointSolution.wsp
- Enable-SPFeature –Identity YourFeatureNameOrGuid –url http://YourSharePointWebApp
- Disable-SPFeature –Identity YourFeatureNameOrGuid –url http://YourSharePointWebApp
- Start-SPAdminJob -Verbose
- New-SPUser – UserAlias ‘YourUserOrGroupName’–displayname ‘Your Display Name’ –web YourSPSite
Here is the complete list of stsadm command and their related PowerShell command: Click HERE
Thursday, April 7, 2011
Generate Test Data - VB.net Class For Test Data Generation
Here is the VB.net code to generate your test data
Download VB.net Code project Enjoy Green Coding @ Code4Green.com
Generate Test Data - CSharp Class For Test Data Generation
Here is the C# code for Test Data generation,

Download Code from Here Enjoy Green coding @ Code4Green.com
Thursday, March 31, 2011
Sharepoint Code Generation Tool
Code4Green.com has launched its Sharepoint Code Generation Tool Yesterday. Now Sharepoint developers can create Feature , Fields, Content Types, and Document Library using this tool. Visual Webparts is already available in code4green under ASP.net Category - It generates ascx control and ascx.cs file for given fields. You can Generate Sharepoint Features(i.e. List, Fields, Content Type, Doc. Library) using Code4green's Sharepoint Code Generation Tool. Please give you feedback and if you need any specific feature in sharepoint code generation, please feel to write me on Info@Code4green.com Enjoy Green Coding.... :)
Thursday, March 17, 2011
Copy and Paste of Script from Web page is becoming single line text
Hi,
I have been asked so many times with the development groups that they can not copy and paste the syntax code from some web pages with indentation.
Issue If a Syntax is in HTML's Code element, than simple copy and paste will paste as a single line text (in notepad or Visual Studio IDE). So, It will remove all the indentation also.
Solution: Copy the code from web page and paste into Excel sheet. It will keep indentation and color coding for you and then after that copy from excel and paste to your IDE.
Sample: Try to copy Syntax code from http://stackoverflow.com/questions/1404513/random-background-image-for-div page, and paste into notepad or IDE. It will become a single line of code.
But if you will copy and paste into excel and again copy from excel than paste into notepad or IDE than if will come into multiple lines with indentation.
Enjoy your Green Code @ code4green.com
I have been asked so many times with the development groups that they can not copy and paste the syntax code from some web pages with indentation.
Issue If a Syntax is in HTML's Code element, than simple copy and paste will paste as a single line text (in notepad or Visual Studio IDE). So, It will remove all the indentation also.
Solution: Copy the code from web page and paste into Excel sheet. It will keep indentation and color coding for you and then after that copy from excel and paste to your IDE.
Sample: Try to copy Syntax code from http://stackoverflow.com/questions/1404513/random-background-image-for-div page, and paste into notepad or IDE. It will become a single line of code.
But if you will copy and paste into excel and again copy from excel than paste into notepad or IDE than if will come into multiple lines with indentation.
Enjoy your Green Code @ code4green.com
Monday, February 14, 2011
What is a distribution group? Why it throws HTTP status 401: Unauthorized Error in Sharepoint
What is a distribution group?
Distribution group is a sharepoint goup which is used for distributing emails to more than one people. whenevenr we want to relay a email message for a group, than we need a distribution group. It need a email id for distribution group, and when we creates a distribution group in sharepoint than automatically a AD group is created.
In MOSS 2007, AD's Distribution group can not be added into people & groups. So MOSS 2007 has added this feature to link AD's DIstribution group with SP. Now you can create a distribution group in Sharepoint and it will creates group in AD.
There are three different places you can find for disribution group in sharepoint:
1. Central Admin (Central Administration > Operations > Incoming E-Mail Settings )
Configure Incoming E-Mail Settings
Set Enable sites on this server to receive e-mail? Yes, and Settings mode: Advanced
Incoming E-Mail Server Display Address
2. under Central adminitrator > Topology and Services, click on Approve/reject distribution groups. it will show view for approved distribution group.
create a new view for ViewAll and don't put any filter. ViewAll will show all the Dtribution groups. based on their status select group which is in pending stage. right click and click on approve.
3.go to people & users and go to groups & select a group. once group is selected than go to settings > group settings. In "E-Mail Distribution List" section, select yes for Create an e-mail distribution group for this group? , and then give the name for group. Click OK.
4.Create document library/list to recieve a incoming email - Create new document library/list. while creating the new list/lib a. select Allow this document library to receive e-mail? Yes, b. Type the email address under Incoming E-Mail section.
If you don't have proper securty at AD for sharepoint account than you will get these errors:
In Central Admin
Object reference not set to an instance of an object. at Microsoft.SharePoint.EmailIntegration.SPEWSApprovalManager.ExecuteJobInAD(Int32 listItemID)
at Microsoft.SharePoint.ApplicationPages.DMSCmdPage.BtnOk_Click(Object sender, EventArgs e)
at System.Web.UI.WebControls.Button.OnClick(EventArgs e)
at System.Web.UI.WebControls.Button.RaisePostBackEvent(String eventArgument)
at System.Web.UI.WebControls.Button.System.Web.UI.IPostBackEventHandler.RaisePostBackEvent(String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(IPostBackEventHandler sourceControl, String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(NameValueCollection postData)
at System.Web.UI.Page.ProcessRequestMain(Boolean includeStagesBeforeAsyncPoint, Boolean includeStagesAfterAsyncPoint)
Troubleshoot issues with Windows SharePoint Services.
------------------------------------------------------------------------------------------------
While Change Group Settings (people & group > groups > select your group > settings > group settings)
The request failed with HTTP status 401: Unauthorized. at System.Web.Services.Protocols.SoapHttpClientProtocol.ReadResponse(SoapClientMessage message, WebResponse response, Stream responseStream, Boolean asyncCall)
at System.Web.Services.Protocols.SoapHttpClientProtocol.Invoke(String methodName, Object[] parameters)
at Microsoft.SharePoint.DirectorySoap.SPDirectoryManagementProxy.CreateDistributionGroup(String Alias, String Name, String Description, String ContactCN, RequestInfo Info, DistributionGroupFlags Flags)
at Microsoft.SharePoint.SPGroup.CreateDMS(String dlAlias, String friendlyName, String description, String[] members, String requestor, String justification, Int32& jobId)
--------------------------------------------------------------------------------------------
Setup groups
Distribution group could not be updated because of the following error: The following error has occurred while attempting to contact the Directory Management Service: The request failed with HTTP status 401: Unauthorized.
I will keep posting with my findings.
Enjoy Green coding at www.code4green.com
Hussain Naqvi
Distribution group is a sharepoint goup which is used for distributing emails to more than one people. whenevenr we want to relay a email message for a group, than we need a distribution group. It need a email id for distribution group, and when we creates a distribution group in sharepoint than automatically a AD group is created.
In MOSS 2007, AD's Distribution group can not be added into people & groups. So MOSS 2007 has added this feature to link AD's DIstribution group with SP. Now you can create a distribution group in Sharepoint and it will creates group in AD.
There are three different places you can find for disribution group in sharepoint:
1. Central Admin (Central Administration > Operations > Incoming E-Mail Settings )
Configure Incoming E-Mail Settings
Set Enable sites on this server to receive e-mail? Yes, and Settings mode: Advanced
Incoming E-Mail Server Display Address
2. under Central adminitrator > Topology and Services, click on Approve/reject distribution groups. it will show view for approved distribution group.
create a new view for ViewAll and don't put any filter. ViewAll will show all the Dtribution groups. based on their status select group which is in pending stage. right click and click on approve.
3.go to people & users and go to groups & select a group. once group is selected than go to settings > group settings. In "E-Mail Distribution List" section, select yes for Create an e-mail distribution group for this group? , and then give the name for group. Click OK.
4.Create document library/list to recieve a incoming email - Create new document library/list. while creating the new list/lib a. select Allow this document library to receive e-mail? Yes, b. Type the email address under Incoming E-Mail section.
If you don't have proper securty at AD for sharepoint account than you will get these errors:
In Central Admin
Object reference not set to an instance of an object. at Microsoft.SharePoint.EmailIntegration.SPEWSApprovalManager.ExecuteJobInAD(Int32 listItemID)
at Microsoft.SharePoint.ApplicationPages.DMSCmdPage.BtnOk_Click(Object sender, EventArgs e)
at System.Web.UI.WebControls.Button.OnClick(EventArgs e)
at System.Web.UI.WebControls.Button.RaisePostBackEvent(String eventArgument)
at System.Web.UI.WebControls.Button.System.Web.UI.IPostBackEventHandler.RaisePostBackEvent(String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(IPostBackEventHandler sourceControl, String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(NameValueCollection postData)
at System.Web.UI.Page.ProcessRequestMain(Boolean includeStagesBeforeAsyncPoint, Boolean includeStagesAfterAsyncPoint)
Troubleshoot issues with Windows SharePoint Services.
------------------------------------------------------------------------------------------------
While Change Group Settings (people & group > groups > select your group > settings > group settings)
The request failed with HTTP status 401: Unauthorized. at System.Web.Services.Protocols.SoapHttpClientProtocol.ReadResponse(SoapClientMessage message, WebResponse response, Stream responseStream, Boolean asyncCall)
at System.Web.Services.Protocols.SoapHttpClientProtocol.Invoke(String methodName, Object[] parameters)
at Microsoft.SharePoint.DirectorySoap.SPDirectoryManagementProxy.CreateDistributionGroup(String Alias, String Name, String Description, String ContactCN, RequestInfo Info, DistributionGroupFlags Flags)
at Microsoft.SharePoint.SPGroup.CreateDMS(String dlAlias, String friendlyName, String description, String[] members, String requestor, String justification, Int32& jobId)
--------------------------------------------------------------------------------------------
Setup groups
Distribution group could not be updated because of the following error: The following error has occurred while attempting to contact the Directory Management Service: The request failed with HTTP status 401: Unauthorized.
I will keep posting with my findings.
Enjoy Green coding at www.code4green.com
Hussain Naqvi
Thursday, January 27, 2011
Get detailed sharepoint error - i.e. File Not Found. at Microsoft.SharePoint.Library
Here is the best way to find out the detailed error of sharepoint.
I was strugling with sharepoint errors for a long time and I have tried lots of options(trace log, log file, event viewer, etc) but nothing has goiven me the exact error.
Finally I got the very good tool which is giving detailed error, and it throw error on scree as soon as any Moss error occured on moss server.
Here is the error I was getting and I tried alot to find out that what is the meaning of this error or which file or template I am missing. but you can not get info from most of general log resources.
Error:
File Not Found. at Microsoft.SharePoint.Library.SPRequestInternalClass.ApplyWebTemplate(String bstrUrl, String& bstrWebTemplate, Int32& plWebTemplateId)
at Microsoft.SharePoint.Library.SPRequest.ApplyWebTemplate(String bstrUrl, String& bstrWebTemplate, Int32& plWebTemplateId)
at Microsoft.SharePoint.SPWeb.ApplyWebTemplate(String strWebTemplate)
at Microsoft.SharePoint.ApplicationPages.TemplatePickerUtil.ApplyWebTemplateAndRedirect(SPWeb Web, String strWebTemplate, Boolean bSharedNav, Boolean bOnTopNav, Boolean bOnQuickLaunch, Page page)
at Microsoft.SharePoint.ApplicationPages.NewSubwebPage.BtnCreateSubweb_Click(Object sender, EventArgs e)
at System.Web.UI.WebControls.Button.OnClick(EventArgs e)
at System.Web.UI.WebControls.Button.RaisePostBackEvent(String eventArgument)
at System.Web.UI.WebControls.Button.System.Web.UI.IPostBackEventHandler.RaisePostBackEvent(String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(IPostBackEventHandler sourceControl, String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(NameValueCollection postData)
at System.Web.UI.Page.ProcessRequestMain(Boolean includeStagesBeforeAsyncPoint, Boolean includeStagesAfterAsyncPoint)
Finally I got the SPTraceViewer on codeplex (http://sptraceview.codeplex.com/). this SPTraceView does analyses in real time the trace messages coming from all MOSS components and it prompt you on machine.
See the attached screen, How detaled error it is giving for the same error message I have posted above.

Enjoy SPTraceViewer and once again thanks for the codeplex's developer for making such a nice tool.
Enjoy GreenCoding @ www.code4green.com
I was strugling with sharepoint errors for a long time and I have tried lots of options(trace log, log file, event viewer, etc) but nothing has goiven me the exact error.
Finally I got the very good tool which is giving detailed error, and it throw error on scree as soon as any Moss error occured on moss server.
Here is the error I was getting and I tried alot to find out that what is the meaning of this error or which file or template I am missing. but you can not get info from most of general log resources.
Error:
File Not Found. at Microsoft.SharePoint.Library.SPRequestInternalClass.ApplyWebTemplate(String bstrUrl, String& bstrWebTemplate, Int32& plWebTemplateId)
at Microsoft.SharePoint.Library.SPRequest.ApplyWebTemplate(String bstrUrl, String& bstrWebTemplate, Int32& plWebTemplateId)
at Microsoft.SharePoint.SPWeb.ApplyWebTemplate(String strWebTemplate)
at Microsoft.SharePoint.ApplicationPages.TemplatePickerUtil.ApplyWebTemplateAndRedirect(SPWeb Web, String strWebTemplate, Boolean bSharedNav, Boolean bOnTopNav, Boolean bOnQuickLaunch, Page page)
at Microsoft.SharePoint.ApplicationPages.NewSubwebPage.BtnCreateSubweb_Click(Object sender, EventArgs e)
at System.Web.UI.WebControls.Button.OnClick(EventArgs e)
at System.Web.UI.WebControls.Button.RaisePostBackEvent(String eventArgument)
at System.Web.UI.WebControls.Button.System.Web.UI.IPostBackEventHandler.RaisePostBackEvent(String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(IPostBackEventHandler sourceControl, String eventArgument)
at System.Web.UI.Page.RaisePostBackEvent(NameValueCollection postData)
at System.Web.UI.Page.ProcessRequestMain(Boolean includeStagesBeforeAsyncPoint, Boolean includeStagesAfterAsyncPoint)
Finally I got the SPTraceViewer on codeplex (http://sptraceview.codeplex.com/). this SPTraceView does analyses in real time the trace messages coming from all MOSS components and it prompt you on machine.
See the attached screen, How detaled error it is giving for the same error message I have posted above.

Enjoy SPTraceViewer and once again thanks for the codeplex's developer for making such a nice tool.
Enjoy GreenCoding @ www.code4green.com
Monday, January 24, 2011
Single-Quote('), double-quote ("), and Tilde (~) keys not recognizing in first hit
Hi,
I have seen this issue in some of the laptops, and the issue is that Single-Quote('), double-quote ("), and Tilde (~) keys not recognizing in first hit. I have searched over the web than I have found that lots of people are facing the same problem.
SO I thought I can write a blog for this issue.
My Laptop's configuration is:
OS - Windows 7 Home Premium, Brand- Acer, Model - Aspire 5741, Processor - Intel Core i3 M350
Problem - From the first day onward I am having this issue. First I was pressing twice these keys and in 2nd time it was writing the 2 characters(',",~).
Reason: Still I am looking the reason. Once I will have that I will post here.
Resolution: I have found the workaround. Keep typing as you do normal typing. So if you hit the key(which has the problem) it will not show at that time, but as soon as you press different key, it will show both typed characters.
i.e. I am typing with the same laptop which has this issue, and for test I have typed the following: "Human Resources". I have typed normally without any issue and it typed correctly.
The only issue I am having in this way: Space bar is not getting recognised in first hit after these keys(",',and ~).
I will keep doing more research and try to contact with Acer with this issue. Once I will have more info I will post here.
Please post your reply if you have the same issue.
Enjoy Green Coding @ www.code4green.com
I have seen this issue in some of the laptops, and the issue is that Single-Quote('), double-quote ("), and Tilde (~) keys not recognizing in first hit. I have searched over the web than I have found that lots of people are facing the same problem.
SO I thought I can write a blog for this issue.
My Laptop's configuration is:
OS - Windows 7 Home Premium, Brand- Acer, Model - Aspire 5741, Processor - Intel Core i3 M350
Problem - From the first day onward I am having this issue. First I was pressing twice these keys and in 2nd time it was writing the 2 characters(',",~).
Reason: Still I am looking the reason. Once I will have that I will post here.
Resolution: I have found the workaround. Keep typing as you do normal typing. So if you hit the key(which has the problem) it will not show at that time, but as soon as you press different key, it will show both typed characters.
i.e. I am typing with the same laptop which has this issue, and for test I have typed the following: "Human Resources". I have typed normally without any issue and it typed correctly.
The only issue I am having in this way: Space bar is not getting recognised in first hit after these keys(",',and ~).
I will keep doing more research and try to contact with Acer with this issue. Once I will have more info I will post here.
Please post your reply if you have the same issue.
Enjoy Green Coding @ www.code4green.com
Subscribe to:
Posts (Atom)